mirror of https://github.com/01-edu/public.git
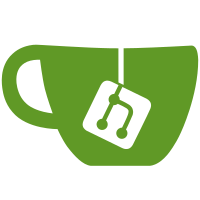
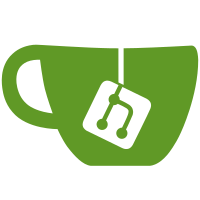
3 changed files with 114 additions and 0 deletions
@ -0,0 +1,36 @@
|
||||
## GoodbyeMars |
||||
|
||||
### Instructions |
||||
|
||||
In a file named `GoodbyeMars.java` write a function `goodbyeMars` that returns the string 'Goodbye Mars !'. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class GoodbyeMars { |
||||
public static String goodbyeMars() { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible `ExerciseRunner.java` to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(GoodbyeMars.goodbyeMars()); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Goodbye Mars ! |
||||
$ |
||||
``` |
@ -0,0 +1,36 @@
|
||||
## SystemLogger |
||||
|
||||
### Instructions |
||||
|
||||
In a file named `SystemLog.java` write a function `systemLog` that takes a String message as a parameter and returns the given message concatenated with 'System Log: ' |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class SystemLog { |
||||
public static String systemLog(String message) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible `ExerciseRunner.java` to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(SystemLog.systemLog("message")); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
System Log: message |
||||
$ |
||||
``` |
@ -0,0 +1,42 @@
|
||||
## UniversalGreeting |
||||
|
||||
### Instructions |
||||
|
||||
In a file named `UniversalGreeting.java` write a function `greeting` that takes a String language as a parameter and returns a greeting message based on the language as follows: |
||||
|
||||
`FR`: `Bonjour comment allez-vous?` |
||||
`EN`: `Hello, How are you?` |
||||
`ES`: `Hola, cómo estás?` |
||||
|
||||
and return an empty string in any other case. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class UniversalGreeting { |
||||
public static String greeting(String message) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible `ExerciseRunner.java` to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(UniversalGreeting.greeting("EN")); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Hello, How are you? |
||||
$ |
||||
``` |
Loading…
Reference in new issue