mirror of https://github.com/01-edu/public.git
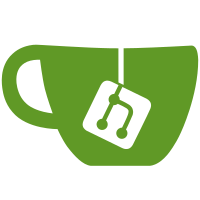
3 changed files with 113 additions and 0 deletions
@ -0,0 +1,36 @@
|
||||
## HelloWorld |
||||
|
||||
### Instructions |
||||
|
||||
In a file named `HelloWorld.java` write a function `helloWorld` that returns the string 'Hello World !'. |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class HelloWorld { |
||||
public static String helloWorld() { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(HelloWorld.helloWorld()); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Hello World ! |
||||
$ |
||||
``` |
@ -0,0 +1,36 @@
|
||||
## SystemLogger |
||||
|
||||
### Instructions |
||||
|
||||
In a file named `SystemLog.java` write a function `systemLog` that takes a String message as a parameter and returns the given message concatenated with 'System Log: ' |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class SystemLog { |
||||
public static String systemLog(String message) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(SystemLog.systemLog('message')); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
System Log: message |
||||
$ |
||||
``` |
@ -0,0 +1,41 @@
|
||||
## UniversalGreeting |
||||
|
||||
### Instructions |
||||
|
||||
In a file named `UniversalGreeting.java` write a function `greeting` that take a String language as parametre and return a greating message based on the language as follows: |
||||
|
||||
FR : Bonjour comment allez-vous |
||||
EN : Hello, How are you |
||||
ES : Hola, cómo estás |
||||
|
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class UniversalGreeting { |
||||
public static String greeting(String message) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible ExerciseRunner.java to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(UniversalGreeting.greeting('EN')); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
Hello, How are you |
||||
$ |
||||
``` |
Loading…
Reference in new issue